Variable and Data Types_2:data type & sizes, variable names, declaration, statements
- Apurba Paul
- Jan 10, 2020
- 10 min read
What is a Variable?
A variable is an identifier which is used to store some value. Constants can never change at the time of execution. Variables can change during the execution of a program and update the value stored inside it.
A single variable can be used at multiple locations in a program. A variable name must be meaningful. It should represent the purpose of the variable.
Example:
Height, age, are the meaningful variables that represent the purpose it is being used for.
Height variable can be used to store a height value.
Age variable can be used to store the age of a person.
A variable must be declared first before it is used somewhere inside the program. A variable name is formed using characters, digits and an underscore.
Following are the rules that must be followed while creating a variable:
A variable name should consist of only characters, digits and an underscore.
A variable name should not begin with a number.
A variable name should not consist of whitespace.
A variable name should not consist of a keyword.
'C' is a case sensitive language that means a variable named 'age' and 'AGE' are different.
Following are the examples of valid variable names in a 'C' program:
height
HEIGHT
_height
_height1
My_name
Following are the examples of invalid variable names in a 'C' program:
1height
Hei$ght
My name
For example, we declare an integer variable my_variable and assign it the value 48:
int my_variable;
my_variable = 48;
By the way, we can both declare and initialize (assign an initial value) a variable in a single statement:
int my_variable = 48;
Data types
'C' provides various data types to make it easy for a programmer to select a suitable data type as per the requirements of an application.
Following are the three data types:
Primitive data types
Derived data types
User-defined data types
There are five primary fundamental data types,
int for integer data
char for character data
float for floating-point numbers
double for double-precision floating-point numbers
void
Array, functions, pointers, structures are derived data types. 'C' language provides more extended versions of the above mentioned primary data types. Each data type differs from one another in size and range.
Integer Types
The following table provides the details of standard integer types with their storage sizes and value ranges −
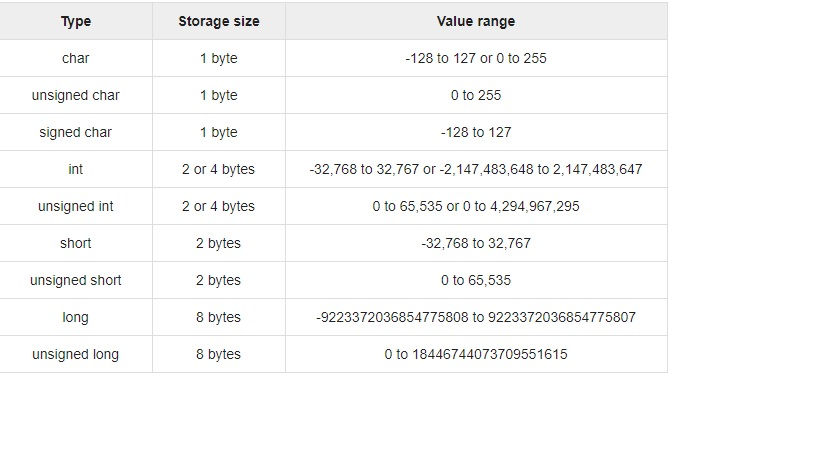
To get the exact size of a type or a variable on a particular platform, you can use the sizeof operator. The expressions sizeof(type) yields the storage size of the object or type in bytes. Given below is an example to get the size of various type on a machine using different constant defined in limits.h header file −
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
#include <float.h>
int main(int argc, char** argv) {
printf("CHAR_BIT : %d\n", CHAR_BIT);
printf("CHAR_MAX : %d\n", CHAR_MAX);
printf("CHAR_MIN : %d\n", CHAR_MIN);
printf("INT_MAX : %d\n", INT_MAX);
printf("INT_MIN : %d\n", INT_MIN);
printf("LONG_MAX : %ld\n", (long) LONG_MAX);
printf("LONG_MIN : %ld\n", (long) LONG_MIN);
printf("SCHAR_MAX : %d\n", SCHAR_MAX);
printf("SCHAR_MIN : %d\n", SCHAR_MIN);
printf("SHRT_MAX : %d\n", SHRT_MAX);
printf("SHRT_MIN : %d\n", SHRT_MIN);
printf("UCHAR_MAX : %d\n", UCHAR_MAX);
printf("UINT_MAX : %u\n", (unsigned int) UINT_MAX);
printf("ULONG_MAX : %lu\n", (unsigned long) ULONG_MAX);
printf("USHRT_MAX : %d\n", (unsigned short) USHRT_MAX);
return 0;
}
When you compile and execute the above program, it produces the following result on Linux −
CHAR_BIT : 8
CHAR_MAX : 127
CHAR_MIN : -128
INT_MAX : 2147483647
INT_MIN : -2147483648
LONG_MAX : 9223372036854775807
LONG_MIN : -9223372036854775808
SCHAR_MAX : 127
SCHAR_MIN : -128
SHRT_MAX : 32767
SHRT_MIN : -32768
UCHAR_MAX : 255
UINT_MAX : 4294967295
ULONG_MAX : 18446744073709551615
USHRT_MAX : 65535
Floating-Point Types
The following table provide the details of standard floating-point types with storage sizes and value ranges and their precision −
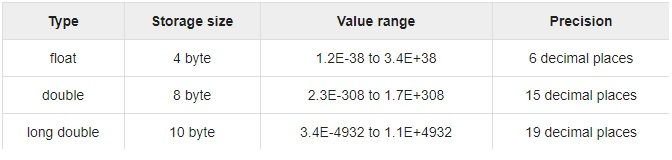
The header file float.h defines macros that allow you to use these values and other details about the binary representation of real numbers in your programs. The following example prints the storage space taken by a float type and its range values −
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
#include <float.h>
int main(int argc, char** argv) {
printf("Storage size for float : %d \n", sizeof(float));
printf("FLT_MAX : %g\n", (float) FLT_MAX);
printf("FLT_MIN : %g\n", (float) FLT_MIN);
printf("-FLT_MAX : %g\n", (float) -FLT_MAX);
printf("-FLT_MIN : %g\n", (float) -FLT_MIN);
printf("DBL_MAX : %g\n", (double) DBL_MAX);
printf("DBL_MIN : %g\n", (double) DBL_MIN);
printf("-DBL_MAX : %g\n", (double) -DBL_MAX);
printf("Precision value: %d\n", FLT_DIG );
return 0;
}
When you compile and execute the above program, it produces the following result on Linux −
Storage size for float : 4
FLT_MAX : 3.40282e+38
FLT_MIN : 1.17549e-38
-FLT_MAX : -3.40282e+38
-FLT_MIN : -1.17549e-38
DBL_MAX : 1.79769e+308
DBL_MIN : 2.22507e-308
-DBL_MAX : -1.79769e+308
Precision value: 6
The void Type
The void type specifies that no value is available. It is used in three kinds of situations −
Function returns as void
There are various functions in C which do not return any value or you can say they return void. A function with no return value has the return type as void. For example, void exit (int status);
Function arguments as void
There are various functions in C which do not accept any parameter. A function with no parameter can accept a void. For example, int rand(void);
Pointers to void
A pointer of type void * represents the address of an object, but not its type. For example, a memory allocation function void *malloc( size_t size ); returns a pointer to void which can be casted to any data type.
Variable Declaration in C
A variable declaration provides assurance to the compiler that there exists a variable with the given type and name so that the compiler can proceed for further compilation without requiring the complete detail about the variable. A variable definition has its meaning at the time of compilation only, the compiler needs actual variable definition at the time of linking the program.
A variable declaration is useful when you are using multiple files and you define your variable in one of the files which will be available at the time of linking of the program. You will use the keyword extern to declare a variable at any place. Though you can declare a variable multiple times in your C program, it can be defined only once in a file, a function, or a block of code.
Example
Try the following example, where variables have been declared at the top, but they have been defined and initialized inside the main function −
#include <stdio.h> // Variable declaration: extern int a, b; extern int c; extern float f; int main () { /* variable definition: */ int a, b; int c; float f; /* actual initialization */ a = 10; b = 20; c = a + b; printf("value of c : %d \n", c); f = 70.0/3.0; printf("value of f : %f \n", f); return 0; }
When the above code is compiled and executed, it produces the following result −
value of c : 30 value of f : 23.333334
The same concept applies on function declaration where you provide a function name at the time of its declaration and its actual definition can be given anywhere else. For example −
// function declaration int func(); int main() { // function call int i = func(); } // function definition int func() { return 0; }
Lvalues and Rvalues in C
There are two kinds of expressions in C −
lvalue − Expressions that refer to a memory location are called "lvalue" expressions. An lvalue may appear as either the left-hand or right-hand side of an assignment.
rvalue − The term rvalue refers to a data value that is stored at some address in memory. An rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side.
Take a look at the following valid and invalid statements −
int g = 20; // valid statement
10 = 20; // invalid statement; would generate compile-time error
Defining Constants
There are two simple ways in C to define constants −
Using #define preprocessor.
Using const keyword.
The #define Preprocessor
Given below is the form to use #define preprocessor to define a constant −
#define identifier value
The following example explains it in detail −
#include <stdio.h>
#define LENGTH 10
#define WIDTH 5
#define NEWLINE '\n'
int main() {
int area;
area = LENGTH * WIDTH;
printf("value of area : %d", area);
printf("%c", NEWLINE);
return 0;
}
When the above code is compiled and executed, it produces the following result −
value of area : 50
The const Keyword
You can use const prefix to declare constants with a specific type as follows −
const type variable = value;
The following example explains it in detail −
#include <stdio.h>
int main() {
const int LENGTH = 10;
const int WIDTH = 5;
const char NEWLINE = '\n';
int area;
area = LENGTH * WIDTH;
printf("value of area : %d", area);
printf("%c", NEWLINE);
return 0;
}
When the above code is compiled and executed, it produces the following result −
value of area : 50
Note that it is a good programming practice to define constants in CAPITALS.
MCQ on Variables
1. Which is valid C expression?
a) int my_num = 100,000;
b) int my_num = 100000;
c) int my num = 1000;
d) int $my_num = 10000;
2. What will be the output of the following C code?
#include <stdio.h>
int main()
{
printf("Hello World! %d \n", x);
return 0;
}
a) Hello World! x;
b) Hello World! followed by a junk value
c) Compile time error
d) Hello World!
3. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int y = 10000;
int y = 34;
printf("Hello World! %d\n", y);
return 0;
}
a) Compile time error
b) Hello World! 34
c) Hello World! 1000
d) Hello World! followed by a junk value
4. Which of the following is not a valid variable name declaration?
a) float PI = 3.14;
b) double PI = 3.14;
c) int PI = 3.14;
d) #define PI 3.14
5. What will happen if the following C code is executed?
#include <stdio.h>
int main()
{
int main = 3;
printf("%d", main);
return 0;
}
a) It will cause a compile-time error
b) It will cause a run-time error
c) It will run without any error and prints 3
d) It will experience infinite looping
6. What is the problem in following variable declaration?
float 3Bedroom-Hall-Kitchen?;
advertisement
a) The variable name begins with an integer
b) The special character ‘-‘
c) The special character ‘?’
d) All of the mentioned
7. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int ThisIsVariableName = 12;
int ThisIsVariablename = 14;
printf("%d", ThisIsVariablename);
return 0;
}
a) The program will print 12
b) The program will print 14
c) The program will have a runtime error
d) The program will cause a compile-time error due to redeclaration
8. Which of the following cannot be a variable name in C? a) volatile b) true c) friend d) export
MCQ on Data Types and Sizes
1. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int a[5] = {1, 2, 3, 4, 5};
int i;
for (i = 0; i < 5; i++)
if ((char)a[i] == '5')
printf("%d\n", a[i]);
else
printf("FAIL\n");
}
a) The compiler will flag an error
b) The program will compile and print the output 5
c) The program will compile and print the ASCII value of 5
d) The program will compile and print FAIL for 5 times
2. The format identifier ‘%i’ is also used for _____ data type.
a) char
b) int
c) float
d) double
3. Which data type is most suitable for storing a number 65000 in a 32-bit system?
a) signed short
b) unsigned short
c) long
d) int
4. Which of the following is a User-defined data type?
a) typedef int Boolean;
b) typedef enum {Mon, Tue, Wed, Thu, Fri} Workdays;
c) struct {char name[10], int age};
d) all of the mentioned
5. What is the size of an int data type?
a) 4 Bytes
b) 8 Bytes
c) Depends on the system/compiler
d) Cannot be determined
6. What will be the output of the following C code?
#include <stdio.h>
int main()
{
signed char chr;
chr = 128;
printf("%d\n", chr);
return 0;
}
a) 128
b) -128
c) Depends on the compiler
d) None of the mentioned
7. What will be the output of the following C code?
advertisement
#include <stdio.h>
int main()
{
char c;
int i = 0;
FILE *file;
file = fopen("test.txt", "w+");
fprintf(file, "%c", 'a');
fprintf(file, "%c", -1);
fprintf(file, "%c", 'b');
fclose(file);
file = fopen("test.txt", "r");
while ((c = fgetc(file)) != -1)
printf("%c", c);
return 0;
}
a) a
b) Infinite loop
c) Depends on what fgetc returns
d) Depends on the compiler
8. What is short int in C programming? a) The basic data type of C b) Qualifier c) Short is the qualifier and int is the basic data type d) All of the mentioned
1. What will be the output of the following C code?
#include <stdio.h>
int main()
{
enum {ORANGE = 5, MANGO, BANANA = 4, PEACH};
printf("PEACH = %d\n", PEACH);
}
a) PEACH = 3
b) PEACH = 4
c) PEACH = 5
d) PEACH = 6
2. What will be the output of the following C code?
#include <stdio.h>
int main()
{
printf("C programming %s", "Class by\n%s Sanfoundry", "WOW");
}
a)
C programming Class by
WOW Sanfoundry
b) C programming Class by\n%s Sanfoundry c)
C programming Class by
%s Sanfoundry
d) Compilation error
3. In the following code snippet, character pointer str holds a reference to the string ___________
char *str = "Sanfoundry.com\0" "training classes";
a) Sanfoundry.com
b) Sanfoundry.com\0training classes
c) Sanfoundry.comtraining classes
d) Invalid declaration
4. What will be the output of the following C code?
#include <stdio.h>
#define a 10
int main()
{
const int a = 5;
printf("a = %d\n", a);
}
a) a = 5
b) a = 10
c) Compilation error
d) Runtime error
5. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int var = 010;
printf("%d", var);
}
a) 2
b) 8
c) 9
d) 10
6. What will be the output of the following C function?
#include <stdio.h>
enum birds {SPARROW, PEACOCK, PARROT};
enum animals {TIGER = 8, LION, RABBIT, ZEBRA};
int main()
{
enum birds m = TIGER;
int k;
k = m;
printf("%d\n", k);
return 0;
}
a) 0
b) Compile time error
c) 1
d) 8
7. What will be the output of the following C code?
#include <stdio.h>
#define MAX 2
enum bird {SPARROW = MAX + 1, PARROT = SPARROW + MAX};
int main()
{
enum bird b = PARROT;
printf("%d\n", b);
return 0;
}
a) Compilation error
b) 5
c) Undefined value
d) 2
8. What will be the output of the following C code?
#include <stdio.h>
#include <string.h>
int main()
{
char *str = "x";
char c = 'x';
char ary[1];
ary[0] = c;
printf("%d %d", strlen(str), strlen(ary));
return 0;
}
a) 1 1 b) 2 1 c) 2 2 d) 1 (undefined value)
Kommentare