Strings in C
Video Link
In C, a string can be referred to either using a character pointer or as a character array. Strings as character arrays
char str[4] = "GfG"; /*One extra for string terminator*/
/* OR */
char str[4] = {‘G’, ‘f’, ‘G’, '\0'}; /* '\0' is string terminator */
When strings are declared as character arrays, they are stored like other types of arrays in C. For example, if str[] is anauto variablethen string is stored in stack segment, if it’s a global or static variable then stored indata segment, etc.
Strings using character pointers Using character pointer strings can be stored in two ways:
1) Read only string in a shared segment. When a string value is directly assigned to a pointer, in most of the compilers, it’s stored in a read-only block (generally in data segment) that is shared among functions.
char*str = "GfG";
In the above line “GfG” is stored in a shared read-only location, but pointer str is stored in a read-write memory. You can change str to point something else but cannot change value at present str. So this kind of string should only be used when we don’t want to modify string at a later stage in the program.
2) Dynamically allocated in heap segment. Strings are stored like other dynamically allocated things in C and can be shared among functions.
char *str;
int size = 4; /*one extra for ‘\0’*/
str = (char *)malloc(sizeof(char)*size);
*(str+0) = 'G';
*(str+1) = 'f';
*(str+2) = 'G';
*(str+3) = '\0';
Let us see some examples to better understand the above ways to store strings.
Example 1 (Try to modify string) The below program may crash (gives segmentation fault error) because the line *(str+1) = ‘n’ tries to write a read only memory.
int main()
{
char *str;
str = "GfG"; /* Stored in read only part of data segment */
*(str+1) = 'n'; /* Problem: trying to modify read only memory */
getchar();
return 0;
}
Below program works perfectly fine as str[] is stored in writable stack segment.
filter_none
int main()
{
char str[] = "GfG"; /* Stored in stack segment like other auto variables */
*(str+1) = 'n'; /* No problem: String is now GnG */
getchar();
return 0;
}
Below program also works perfectly fine as data at str is stored in writable heap segment.
int main() { int size = 4; /* Stored in heap segment like other dynamically allocated things */ char *str = (char *)malloc(sizeof(char)*size); *(str+0) = 'G'; *(str+1) = 'f'; *(str+2) = 'G'; *(str+3) = '\0'; *(str+1) = 'n'; /* No problem: String is now GnG */ getchar(); return 0; }
Example 2 (Try to return string from a function) The below program works perfectly fine as the string is stored in a shared segment and data stored remains there even after return of getString()
char *getString() { char *str = "GfG"; /* Stored in read only part of shared segment */ /* No problem: remains at address str after getString() returns*/ return str; } int main() { printf("%s", getString()); getchar(); return 0; }
The below program also works perfectly fine as the string is stored in heap segment and data stored in heap segment persists even after the return of getString()
char *getString()
{
int size = 4;
char *str = (char *)malloc(sizeof(char)*size); /*Stored in heap segment*/
*(str+0) = 'G';
*(str+1) = 'f';
*(str+2) = 'G';
*(str+3) = '\0';
/* No problem: string remains at str after getString() returns */
return str;
}
int main()
{
printf("%s", getString());
getchar();
return 0;
}
But, the below program may print some garbage data as string is stored in stack frame of function getString() and data may not be there after getString() returns.
char *getString()
{
char str[] = "GfG"; /* Stored in stack segment */
/* Problem: string may not be present after getSting() returns */
return str;
}
int main()
{
printf("%s", getString());
getchar();
return 0;
}
Arrays in C/C++
Video link
An array in C or C++ is a collection of items stored at contiguous memory locations and elements can be accessed randomly using indices of an array. They are used to store similar type of elements as in the data type must be the same for all elements. They can be used to store collection of primitive data types such as int, float, double, char, etc of any particular type. To add to it, an array in C or C++ can store derived data types such as the structures, pointers etc. Given below is the picturesque representation of an array.
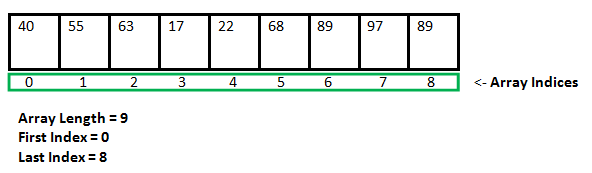
Why do we need arrays?
We can use normal variables (v1, v2, v3, ..) when we have a small number of objects, but if we want to store a large number of instances, it becomes difficult to manage them with normal variables. The idea of an array is to represent many instances in one variable.
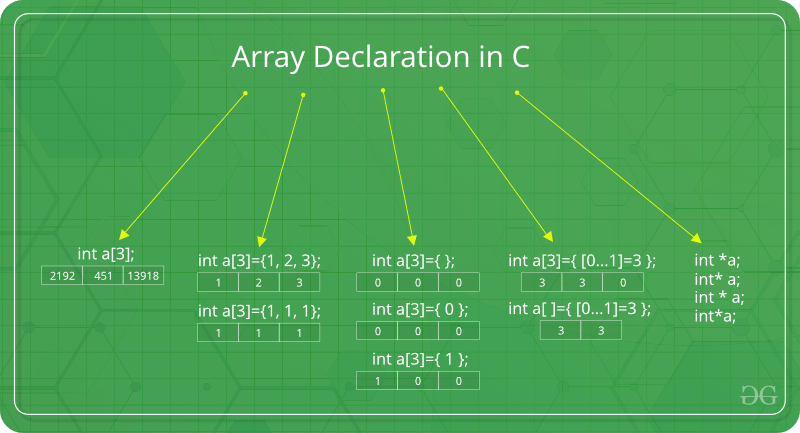
There are various ways in which we can declare an array. It can be done by specifying its type and size, by initializing it or both.
Array declaration by specifying size
// Array declaration by specifying size int arr1[10]; // With recent C/C++ versions, we can also // declare an array of user specified size int n = 10; int arr2[n]; // Array declaration by initializing elements int arr[] = { 10, 20, 30, 40 } // Compiler creates an array of size 4. // above is same as "int arr[4] = {10, 20, 30, 40}" // Array declaration by specifying size and initializing // elements int arr[6] = { 10, 20, 30, 40 } // Compiler creates an array of size 6, initializes first // 4 elements as specified by user and rest two elements as 0. // above is same as "int arr[] = {10, 20, 30, 40, 0, 0}" Advantages of an Array in C/C++:
Random access of elements using array index.
Use of less line of code as it creates a single array of multiple elements.
Easy access to all the elements.
Traversal through the array becomes easy using a single loop.
Sorting becomes easy as it can be accomplished by writing less line of code.
Disadvantages of an Array in C/C++:
Allows a fixed number of elements to be entered which is decided at the time of declaration. Unlike a linked list, an array in C is not dynamic.
Insertion and deletion of elements can be costly since the elements are needed to be managed in accordance with the new memory allocation.
Facts about Array in C/C++:
Accessing Array Elements: Array elements are accessed by using an integer index. Array index starts with 0 and goes till size of array minus 1
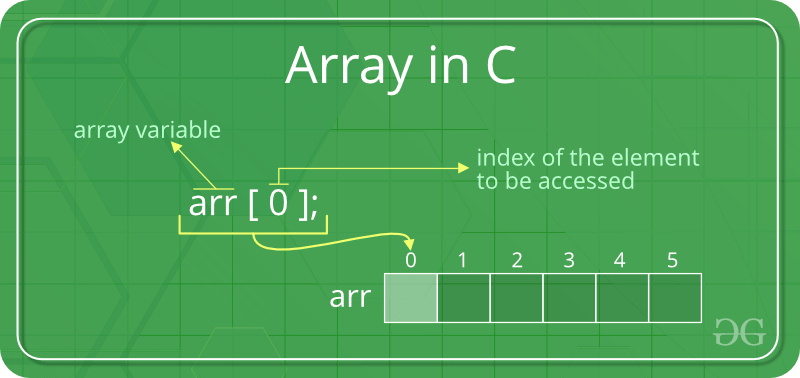
#include <stdio.h> int main() { int arr[5]; arr[0] = 5; arr[2] = -10; arr[3 / 2] = 2; // this is same as arr[1] = 2 arr[3] = arr[0]; printf("%d %d %d %d", arr[0], arr[1], arr[2], arr[3]); return 0; } No Index Out of bound Checking:
There is no index out of bounds checking in C/C++, for example, the following program compiles fine but may produce unexpected output when run.
// This C program compiles fine // as index out of bound // is not checked in C. #include <stdio.h> int main() { int arr[2]; printf("%d ", arr[3]); printf("%d ", arr[-2]); return 0; } In C, it is not compiler error to initialize an array with more elements than the specified size. For example, the below program compiles fine and shows just Warning.
#include <stdio.h> int main() { // Array declaration by initializing it with more // elements than specified size. int arr[2] = { 10, 20, 30, 40, 50 }; return 0; } The elements are stored at contiguous memory locations
Example:
// C program to demonstrate that array elements are stored // contiguous locations #include <stdio.h> int main() { // an array of 10 integers. If arr[0] is stored at // address x, then arr[1] is stored at x + sizeof(int) // arr[2] is stored at x + sizeof(int) + sizeof(int) // and so on. int arr[5], i; printf("Size of integer in this compiler is %lu\n", sizeof(int)); for (i = 0; i < 5; i++) // The use of '&' before a variable name, yields // address of variable. printf("Address arr[%d] is %p\n", i, &arr[i]); return 0; }
Pointers in C/C++ with Examples
Video Link
Pointers are symbolic representation of addresses. They enable programs to simulate call-by-reference as well as to create and manipulate dynamic data structures. It’s general declaration in C/C++ has the format:
Syntax:
datatype *var_name;
int *ptr; //ptr can point to an address which holds int data
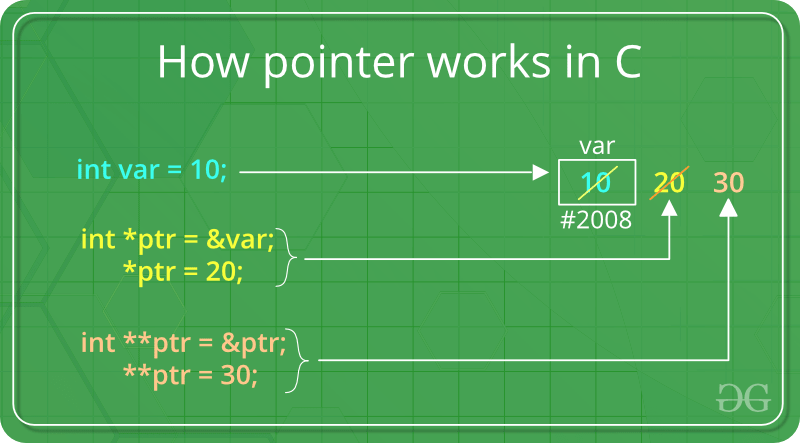
How to use a pointer?
Define a pointer variable
Assigning the address of a variable to a pointer using unary operator (&) which returns the address of that variable.
Accessing the value stored in the address using unary operator (*) which returns the value of the variable located at the address specified by its operand.
The reason we associate data type to a pointer is that it knows how many bytes the data is stored in. When we increment a pointer, we increase the pointer by the size of data type to which it points.
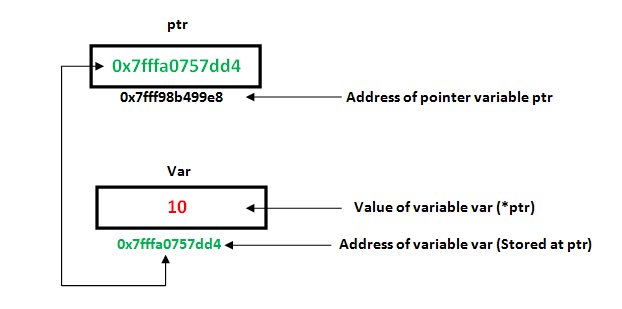
// C++ program to illustrate Pointers in C++ #include <stdio.h> void geeks() { int var = 20; // declare pointer variable int *ptr; // note that data type of ptr and var must be same ptr = &var; // assign the address of a variable to a pointer printf("Value at ptr = %p \n",ptr); printf("Value at var = %d \n",var); printf("Value at *ptr = %d \n", *ptr); } // Driver program int main() { geeks(); } References and Pointers
There are 3 ways to pass C++ arguments to a function:
call-by-value
call-by-reference with pointer argument
call-by-reference with reference argument
// C++ program to illustrate call-by-methods in C++
#include <bits/stdc++.h>
using namespace std;
//Pass-by-Value
int square1(int n)
{
//Address of n in square1() is not the same as n1 in main()
cout << "address of n1 in square1(): " << &n << "\n";
// clone modified inside the function
n *= n;
return n;
}
//Pass-by-Reference with Pointer Arguments
void square2(int *n)
{
//Address of n in square2() is the same as n2 in main()
cout << "address of n2 in square2(): " << n << "\n";
// Explicit de-referencing to get the value pointed-to
*n *= *n;
}
//Pass-by-Reference with Reference Arguments
void square3(int &n)
{
//Address of n in square3() is the same as n3 in main()
cout << "address of n3 in square3(): " << &n << "\n";
// Implicit de-referencing (without '*')
n *= n;
}
void geeks()
{
//Call-by-Value
int n1=8;
cout << "address of n1 in main(): " << &n1 << "\n";
cout << "Square of n1: " << square1(n1) << "\n";
cout << "No change in n1: " << n1 << "\n";
//Call-by-Reference with Pointer Arguments
int n2=8;
cout << "address of n2 in main(): " << &n2 << "\n";
square2(&n2);
cout << "Square of n2: " << n2 << "\n";
cout << "Change reflected in n2: " << n2 << "\n";
//Call-by-Reference with Reference Arguments
int n3=8;
cout << "address of n3 in main(): " << &n3 << "\n";
square3(n3);
cout << "Square of n3: " << n3 << "\n";
cout << "Change reflected in n3: " << n3 << "\n";
}
//Driver program
int main()
{
geeks();
}
Array Name as Pointers
An array name contains the address of first element of the array which acts like constant pointer. It means, the address stored in array name can’t be changed. For example, if we have an array named val then val and &val[0] can be used interchangeably.
// C program to illustrate call-by-methods
#include <stdio.h>
void geeks()
{
//Declare an array
int val[3] = { 5, 10, 20 };
//declare pointer variable
int *ptr;
//Assign the address of val[0] to ptr
// We can use ptr=&val[0];(both are same)
ptr = val ;
printf("Elements of the array are: ");
printf("%d %d %d", ptr[0], ptr[1], ptr[2]);
}
//Driver program
int main()
{
geeks();
}

Pointer Expressions and Pointer Arithmetic
A limited set of arithmetic operations can be performed on pointers which are:
incremented ( ++ )
decremented ( — )
an integer may be added to a pointer ( + or += )
an integer may be subtracted from a pointer ( – or -= )
difference between two pointers (p1-p2)
// C++ program to illustrate Pointer Arithmetic in C++
#include <bits/stdc++.h>
using namespace std;
void geeks()
{
//Declare an array
int v[3] = {10, 100, 200};
//declare pointer variable
int *ptr;
//Assign the address of v[0] to ptr
ptr = v;
for (int i = 0; i < 3; i++)
{
cout << "Value at ptr = " << ptr << "\n";
cout << "Value at *ptr = " << *ptr << "\n";
// Increment pointer ptr by 1
ptr++;
}
}
//Driver program
int main()
{
geeks();
}
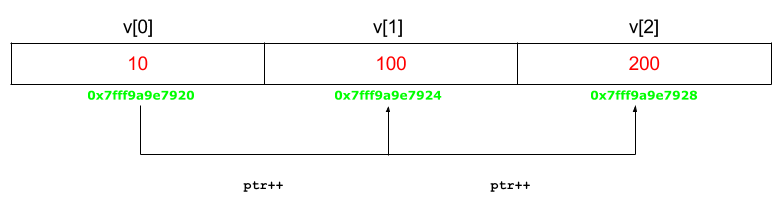
Advanced Pointer Notation
Consider pointer notation for the two-dimensional numeric arrays. consider the following declaration
int nums[2][3] = { { 16, 18, 20 }, { 25, 26, 27 } };
In general, nums[ i ][ j ] is equivalent to *(*(nums+i)+j)

Pointers and String literals
String literals are arrays containing null-terminated character sequences. String literals are arrays of type character plus terminating null-character, with each of the elements being of type const char (as characters of string can’t be modified).
const char * ptr = "geek";
This declares an array with the literal representation for “geek”, and then a pointer to its first element is assigned to ptr. If we imagine that “geek” is stored at the memory locations that start at address 1800, we can represent the previous declaration as:

As pointers and arrays behave in the same way in expressions, ptr can be used to access the characters of string literal. For example:
char x = *(ptr+3);
char y = ptr[3];
Here, both x and y contain k stored at 1803 (1800+3).
Pointers to pointers
In C++, we can create a pointer to a pointer that in turn may point to data or other pointer. The syntax simply requires the unary operator (*) for each level of indirection while declaring the pointer.
char a;
char *b;
char ** c;
a = ’g’;
b = &a;
c = &b;
Here b points to a char that stores ‘g’ and c points to the pointer b.
This is a special type of pointer available in C++ which represents absence of type. void pointers are pointers that point to a value that has no type (and thus also an undetermined length and undetermined dereferencing properties). This means that void pointers have great flexibility as it can point to any data type. There is payoff for this flexibility. These pointers cannot be directly dereferenced. They have to be first transformed into some other pointer type that points to a concrete data type before being dereferenced.
// C++ program to illustrate Void Pointer in C++ #include <bits/stdc++.h> using namespace std; void increase(void *data,int ptrsize) { if(ptrsize == sizeof(char)) { char *ptrchar; //Typecast data to a char pointer ptrchar = (char*)data; //Increase the char stored at *ptrchar by 1 (*ptrchar)++; cout << "*data points to a char"<<"\n"; } else if(ptrsize == sizeof(int)) { int *ptrint; //Typecast data to a int pointer ptrint = (int*)data; //Increase the int stored at *ptrchar by 1 (*ptrint)++; cout << "*data points to an int"<<"\n"; } } void geek() { // Declare a character char c='x'; // Declare an integer int i=10; //Call increase function using a char and int address respectively increase(&c,sizeof(c)); cout << "The new value of c is: " << c <<"\n"; increase(&i,sizeof(i)); cout << "The new value of i is: " << i <<"\n"; } //Driver program int main() { geek(); } Invalid pointers
A pointer should point to a valid address but not necessarily to valid elements (like for arrays). These are called invalid pointers. Uninitialized pointers are also invalid pointers.
int *ptr1;
int arr[10];
int *ptr2 = arr+20;
Here, ptr1 is uninitialized so it becomes an invalid pointer and ptr2 is out of bounds of arr so it also becomes an invalid pointer. (Note: invalid pointers do not necessarily raise compile errors)
Null pointer is a pointer which point nowhere and not just an invalid address. Following are 2 methods to assign a pointer as NULL;
int *ptr1 = 0;
int *ptr2 = NULL;
Comments