Fundamentals and Program Structures
- Apurba Paul
- Apr 14, 2020
- 6 min read
Difference between Call by Value and Call by Reference
Functions can be invoked in two ways: Call by Value or Call by Reference. These two ways are generally differentiated by the type of values passed to them as parameters.
The parameters passed to function are called actual parameters whereas the parameters received by function are called formal parameters.
Call By Value: In this parameter passing method, values of actual parameters are copied to function’s formal parameters and the two types of parameters are stored in different memory locations. So any changes made inside functions are not reflected in actual parameters of caller.
Call by Reference: Both the actual and formal parameters refer to same locations, so any changes made inside the function are actually reflected in actual parameters of caller.
CALL BY VALUECALL BY REFERENCEWhile calling a function, we pass values of variables to it. Such functions are known as “Call By Values”.While calling a function, instead of passing the values of variables, we pass address of variables(location of variables) to the function known as “Call By References.In this method, the value of each variable in calling function is copied into corresponding dummy variables of the called function.In this method, the address of actual variables in the calling function are copied into the dummy variables of the called function.With this method, the changes made to the dummy variables in the called function have no effect on the values of actual variables in the calling function.With this method, using addresses we would have an access to the actual variables and hence we would be able to manipulate them.
// C program to illustrate
// call by value
#include <stdio.h>
// Function Prototype
void swapx(int x, int y);
// Main function
int main()
{
int a = 10, b = 20;
// Pass by Values
swapx(a, b);
printf("a=%d b=%d\n", a, b);
return 0;
}
// Swap functions that swaps
// two values
void swapx(int x, int y)
{
int t;
t = x;
x = y;
y = t;
printf("x=%d y=%d\n", x, y);
}
// C program to illustrate
// Call by Reference
#include <stdio.h>
// Function Prototype
void swapx(int*, int*);
// Main function
int main()
{
int a = 10, b = 20;
// Pass reference
swapx(&a, &b);
printf("a=%d b=%d\n", a, b);
return 0;
}
// Function to swap two variables
// by references
void swapx(int* x, int* y)
{
int t;
t = *x;
*x = *y;
*y = t;
printf("x=%d y=%d\n", *x, *y);
}
Thus actual values of a and b remain unchanged even after exchanging the values of x and y.Thus actual values of a and b get changed after exchanging values of x and y.
In call by values we cannot alter the values of actual variables through function calls.
In call by reference we can alter the values of variables through function calls.
Values of variables are passes by Simple technique.
Pointer variables are necessary to define to store the address values of variables.
Storage Classes in C
Storage Classes are used to describe the features of a variable/function. These features basically include the scope, visibility and life-time which help us to trace the existence of a particular variable during the runtime of a program. C language uses 4 storage classes, namely:
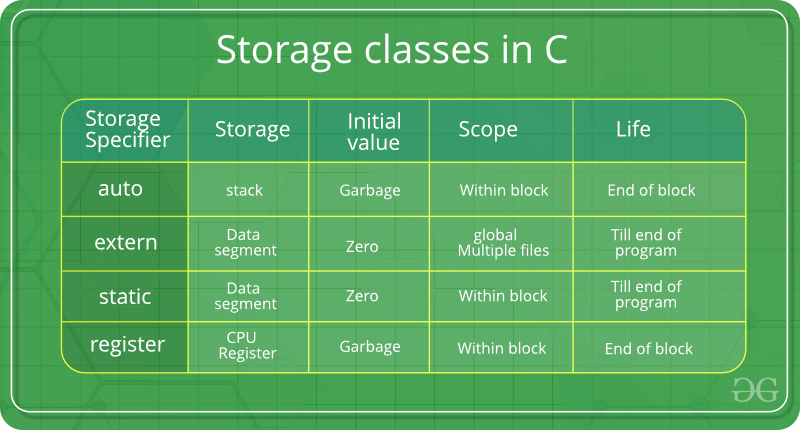
auto: This is the default storage class for all the variables declared inside a function or a block. Hence, the keyword auto is rarely used while writing programs in C language. Auto variables can be only accessed within the block/function they have been declared and not outside them (which defines their scope). Of course, these can be accessed within nested blocks within the parent block/function in which the auto variable was declared. However, they can be accessed outside their scope as well using the concept of pointers given here by pointing to the very exact memory location where the variables resides. They are assigned a garbage value by default whenever they are declared.
2. extern: Extern storage class simply tells us that the variable is defined elsewhere and not within the same block where it is used. Basically, the value is assigned to it in a different block and this can be overwritten/changed in a different block as well. So an extern variable is nothing but a global variable initialized with a legal value where it is declared in order to be used elsewhere. It can be accessed within any function/block. Also, a normal global variable can be made extern as well by placing the ‘extern’ keyword before its declaration/definition in any function/block. This basically signifies that we are not initializing a new variable but instead we are using/accessing the global variable only. The main purpose of using extern variables is that they can be accessed between two different files which are part of a large program. For more information on how extern variables work, have a look at this link.
3. static: This storage class is used to declare static variables which are popularly used while writing programs in C language. Static variables have a property of preserving their value even after they are out of their scope! Hence, static variables preserve the value of their last use in their scope. So we can say that they are initialized only once and exist till the termination of the program. Thus, no new memory is allocated because they are not re-declared. Their scope is local to the function to which they were defined. Global static variables can be accessed anywhere in the program. By default, they are assigned the value 0 by the compiler.
4. register: This storage class declares register variables which have the same functionality as that of the auto variables. The only difference is that the compiler tries to store these variables in the register of the microprocessor if a free register is available. This makes the use of register variables to be much faster than that of the variables stored in the memory during the runtime of the program. If a free register is not available, these are then stored in the memory only. Usually few variables which are to be accessed very frequently in a program are declared with the register keyword which improves the running time of the program. An important and interesting point to be noted here is that we cannot obtain the address of a register variable using pointers.
To specify the storage class for a variable, the following syntax is to be followed:
Syntax:
storage_class var_data_type var_name;
// A C program to demonstrate different storage
// classes
#include <stdio.h>
// declaring the variable which is to be made extern
// an intial value can also be initialized to x
int x;
void autoStorageClass()
{
printf("\nDemonstrating auto class\n\n");
// declaring an auto variable (simply
// writing "int a=32;" works as well)
auto int a = 32;
// printing the auto variable 'a'
printf("Value of the variable 'a'"
" declared as auto: %d\n",
a);
printf("--------------------------------");
}
void registerStorageClass()
{
printf("\nDemonstrating register class\n\n");
// declaring a register variable
register char b = 'G';
// printing the register variable 'b'
printf("Value of the variable 'b'"
" declared as register: %d\n",
b);
printf("--------------------------------");
}
void externStorageClass()
{
printf("\nDemonstrating extern class\n\n");
// telling the compiler that the variable
// z is an extern variable and has been
// defined elsewhere (above the main
// function)
extern int x;
// printing the extern variables 'x'
printf("Value of the variable 'x'"
" declared as extern: %d\n",
x);
// value of extern variable x modified
x = 2;
// printing the modified values of
// extern variables 'x'
printf("Modified value of the variable 'x'"
" declared as extern: %d\n",
x);
printf("--------------------------------");
}
void staticStorageClass()
{
int i = 0;
printf("\nDemonstrating static class\n\n");
// using a static variable 'y'
printf("Declaring 'y' as static inside the loop.\n"
"But this declaration will occur only"
" once as 'y' is static.\n"
"If not, then every time the value of 'y' "
"will be the declared value 5"
" as in the case of variable 'p'\n");
printf("\nLoop started:\n");
for (i = 1; i < 5; i++) {
// Declaring the static variable 'y'
static int y = 5;
// Declare a non-static variable 'p'
int p = 10;
// Incrementing the value of y and p by 1
y++;
p++;
// printing value of y at each iteration
printf("\nThe value of 'y', "
"declared as static, in %d "
"iteration is %d\n",
i, y);
// printing value of p at each iteration
printf("The value of non-static variable 'p', "
"in %d iteration is %d\n",
i, p);
}
printf("\nLoop ended:\n");
printf("--------------------------------");
}
int main()
{
printf("A program to demonstrate"
" Storage Classes in C\n\n");
// To demonstrate auto Storage Class
autoStorageClass();
// To demonstrate register Storage Class
registerStorageClass();
// To demonstrate extern Storage Class
externStorageClass();
// To demonstrate static Storage Class
staticStorageClass();
// exiting
printf("\n\nStorage Classes demonstrated");
return 0;
}
Functions follow the same syntax as given above for variables. Have a look at the following C example for further clarification:
Comments