Doubly Linked List- Part 1
- Apurba Paul
- Aug 8, 2019
- 1 min read
Doubly linked list is a type of linked list in which each node apart from storing its data has two links. The first link points to the previous node in the list and the second link points to the next node in the list. The first node of the list has its previous link pointing to NULL similarly the last node of the list has its next node pointing to NULL.
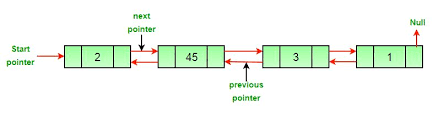
#include<stdio.h>
#include<stdlib.h>
typedef struct mynode
{
struct mynode *lptr;
int info;
struct mynode *rptr;
}Node;
void add_node(int);
void display();
Node *start,*last;
void add_node(int data)
{
Node *newptr=NULL;
newptr=(Node*)malloc(sizeof(Node));
newptr->info=data;
newptr->rptr=NULL;
if(last==NULL)
{
newptr->lptr=NULL;
start=last=newptr;
}
else
{
last->rptr=newptr;
newptr->lptr=last;
last=newptr;
}
}
void display()
{
Node *move;
printf("\n Traversing in the forward direction:\n\n");
move=start;
while(move)
{
printf("%d<->",move->info);
move=move->rptr;
}
printf("NULL");
printf("\n Traversing in the backward direction:\n\n");
move=last;
while(move)
{
printf("%d<->",move->info);
move=move->lptr;
}
printf("NULL");
}
int main()
{
int value;
char choice;
start=last=NULL;
while(1)
{
printf("\n Do you want to insert the node(Y/N)?");
scanf("%c",&choice);
if(choice=='Y'||choice=='y')
{
printf("\n Enter the information of the Node:");
scanf("%d",&value);
add_node(value);
fflush(stdin);
}
else
{
break;
}
}
printf("\n The Doubly Linked List id:\n\n");
display();
return 0;
}
Comments