C Operators & Expressions_2 & 3: type, conversion, precedence and order of evaluation
- Apurba Paul
- Jan 6, 2020
- 7 min read
Type Conversion in C
A type cast is basically a conversion from one type to another. There are two types of type conversion:
Implicit Type Conversion
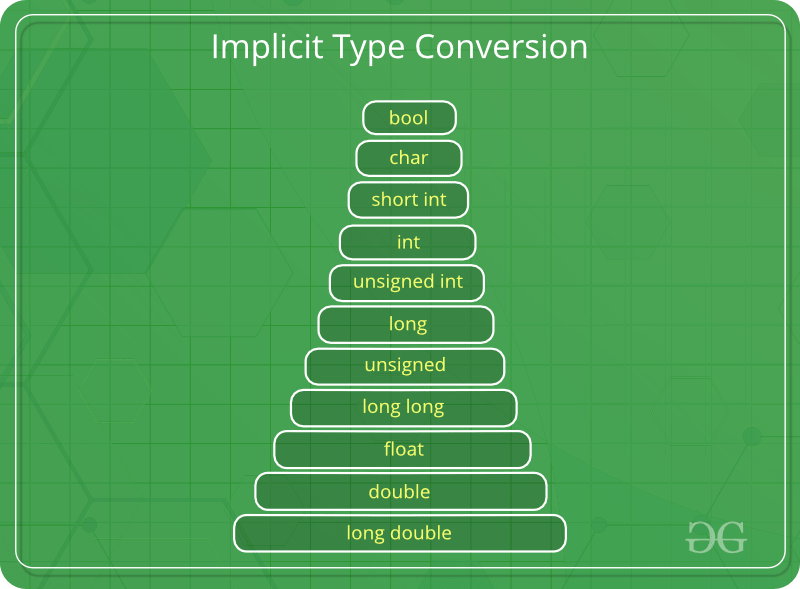
Done by the compiler on its own, without any external trigger from the user.
Generally takes place when in an expression more than one data type is present.
In such condition type conversion (type promotion) takes place to avoid loss of data.
All the data types of the variables are upgraded to the data type of the variable with the largest data type.
It is possible for implicit conversions to lose information, signs can be lost (when signed is implicitly converted to unsigned), and overflow can occur (when long long is implicitly converted to float).
bool -> char -> short int -> int ->
unsigned int -> long -> unsigned ->
long long -> float -> double -> long double
#include<stdio.h>
int main()
{
int x = 10; // integer x
char y = 'a'; // character c
// y implicitly converted to int. ASCII
// value of 'a' is 97
x = x + y;
// x is implicitly converted to float
float z = x + 1.0;
printf("x = %d, z = %f", x, z);
return 0;
}
Explicit Type Conversion

This process is also called type casting and it is user defined. Here the user can type cast the result to make it of a particular data type.
The syntax in C:
(type) expression
Type indicated the data type to which the final result is converted.
#include<stdio.h>
int main()
{
double x = 1.2;
// Explicit conversion from double to int
int sum = (int)x + 1;
printf("sum = %d", sum);
return 0;
}
Advantages of Type Conversion:
This is done to take advantage of certain features of type hierarchies or type representations.
It helps us to compute expressions containing variables of different data types.
Operator Precedence:
Find the link below
Questions:
1. What will be the output of the following C function?
#include <stdio.h>
int main()
{
reverse(1);
}
void reverse(int i)
{
if (i > 5)
exit(0);
printf("%d\n", i);
return reverse(i++);
}
a) 1 2 3 4 5
b) 1 2 3 4
c) Compile time error
d) Stack overflow
2. What will be the output of the following C function?
#include <stdio.h>
void reverse(int i);
int main()
{
reverse(1);
}
void reverse(int i)
{
if (i > 5)
return ;
printf("%d ", i);
return reverse((i++, i));
}
a) 1 2 3 4 5
b) Segmentation fault
c) Compilation error
d) Undefined behaviour
3. In expression i = g() + f(), first function called depends on __________
a) Compiler
b) Associativiy of () operator
c) Precedence of () and + operator
d) Left to write of the expression
4. What will be the final values of i and j in the following C code?
#include <stdio.h>
int x = 0;
int main()
{
int i = (f() + g()) || g();
int j = g() || (f() + g());
}
int f()
{
if (x == 0)
return x + 1;
else
return x - 1;
}
int g()
{
return x++;
}
a) i value is 1 and j value is 1
b) i value is 0 and j value is 0
c) i value is 1 and j value is undefined
d) i and j value are undefined
5. What will be the final values of i and j in the following C code?
#include <stdio.h>
int x = 0;
int main()
{
int i = (f() + g()) | g(); //bitwise or
int j = g() | (f() + g()); //bitwise or
}
int f()
{
if (x == 0)
return x + 1;
else
return x - 1;
}
int g()
{
return x++;
}
a) i value is 1 and j value is 1
b) i value is 0 and j value is 0
c) i value is 1 and j value is undefined
d) i and j value are undefined
6. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 0;
int z = y && (y |= 10);
printf("%d\n", z);
return 0;
}
a) 1
b) 0
c) Undefined behaviour due to order of evaluation
d) 2
7. What will be the output of the following C code?
advertisement
#include <stdio.h>
int main()
{
int x = 2, y = 0;
int z = (y++) ? 2 : y == 1 && x;
printf("%d\n", z);
return 0;
}
a) 0
b) 1
c) 2
d) Undefined behaviour
8. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 0;
int z;
z = (y++, y);
printf("%d\n", z);
return 0;
}
a) 0
b) 1
c) Undefined behaviour
d) Compilation error
9. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 0, l;
int z;
z = y = 1, l = x && y;
printf("%d\n", l);
return 0;
}
a) 0
b) 1
c) Undefined behaviour due to order of evaluation can be different
d) Compilation error
10. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int y = 2;
int z = y +(y = 10);
printf("%d\n", z);
}
a) 12 b) 20 c) 4 d) Either 12 or 20
1. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int b = 5 - 4 + 2 * 5;
printf("%d", b);
}
a) 25
b) -5
c) 11
d) 16
2. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int b = 5 & 4 & 6;
printf("%d", b);
}
a) 5
b) 6
c) 3
d) 4
3. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int b = 5 & 4 | 6;
printf("%d", b);
}
a) 6
b) 4
c) 1
d) 0
4. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int b = 5 + 7 * 4 - 9 * (3, 2);
printf("%d", b);
}
a) 6
b) 15
c) 13
d) 21
5. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int h = 8;
int b = (h++, h++);
printf("%d%d\n", b, h);
}
a) 10 10
b) 10 9
c) 9 10
d) 8 10
6. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int h = 8;
int b = h++ + h++ + h++;
printf("%d\n", h);
}
a) 9
b) 10
c) 12
d) 11
7. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int h = 8;
int b = 4 * 6 + 3 * 4 < 3 ? 4 : 3;
printf("%d\n", b);
}
a) 3
b) 33
c) 34
d) Run time error
8. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int a = 2 + 3 - 4 + 8 - 5 % 4;
printf("%d\n", a);
}
a) 0
b) 8
c) 11
d) 9
9. What will be the output of the following C code?
#include <stdio.h>
void main()
{
char a = '0';
char b = 'm';
int c = a && b || '1';
printf("%d\n", c);
}
a) 0
b) a
c) 1
d) m
10. What will be the output of the following C code?
#include <stdio.h>
void main()
{
char a = 'A';
char b = 'B';
int c = a + b % 3 - 3 * 2;
printf("%d\n", c);
}
a) 65 b) 58 c) 64 d) 59
1. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int b = 5 - 4 + 2 * 5;
printf("%d", b);
}
a) 25
b) -5
c) 11
d) 16
2. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int b = 5 & 4 & 6;
printf("%d", b);
}
a) 5
b) 6
c) 3
d) 4
3. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int b = 5 & 4 | 6;
printf("%d", b);
}
a) 6
b) 4
c) 1
d) 0
4. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int b = 5 + 7 * 4 - 9 * (3, 2);
printf("%d", b);
}
a) 6
b) 15
c) 13
d) 21
5. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int h = 8;
int b = (h++, h++);
printf("%d%d\n", b, h);
}
a) 10 10
b) 10 9
c) 9 10
d) 8 10
6. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int h = 8;
int b = h++ + h++ + h++;
printf("%d\n", h);
}
a) 9 b) 10 c) 12 d) 11
1. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 2;
float f = y + x /= x / y;
printf("%d %f\n", x, f);
return 0;
}
a) 2 4.000000
b) Compile time error
c) 2 3.500000
d) Undefined behaviour
2. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 1, y = 2;
if (x && y == 1)
printf("true\n");
else
printf("false\n");
}
a) true
b) false
c) compile time error
d) undefined behaviour
3. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 1, y = 2;
int z = x & y == 2;
printf("%d\n", z);
}
a) 0
b) 1
c) Compile time error
d) Undefined behaviour
4. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 3, y = 2;
int z = x /= y %= 2;
printf("%d\n", z);
}
a) 1
b) Compile time error
c) Floating point exception
d) Segmentation fault
5. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 3, y = 2;
int z = x << 1 > 5;
printf("%d\n", z);
}
a) 1
b) 0
c) 3
d) Compile time error
6. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 3; //, y = 2;
const int *p = &x;
*p++;
printf("%d\n", *p);
}
a) Increment of read-only location compile error b) 4 c) Some garbage value d) Undefined behaviour
Kommentarer