An operator is a symbol that tells the compiler to perform specific mathematical or logical functions. C language is rich in built-in operators and provides the following types of operators −
Arithmetic Operators
Relational Operators
Logical Operators
Bitwise Operators
Assignment Operators
Misc Operators
We will, in this chapter, look into the way each operator works.
Arithmetic Operators
The following table shows all the arithmetic operators supported by the C language. Assume variable A holds 10 and variable B holds 20 then −
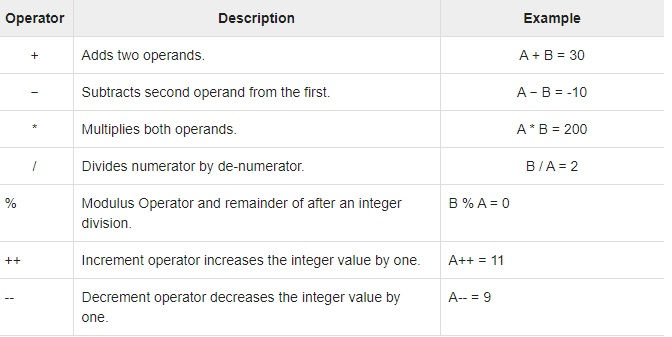
Relational Operators
The following table shows all the relational operators supported by C. Assume variable A holds 10 and variable B holds 20 then −
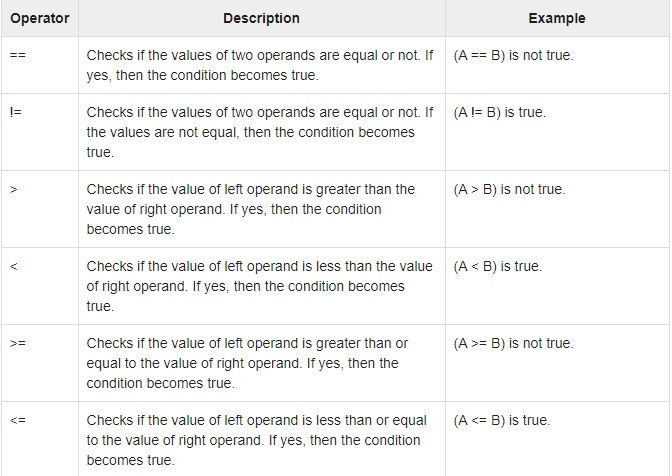
Logical Operators
The following table shows all the logical operators supported by C language. Assume variable A holds 1 and variable B holds 0, then −
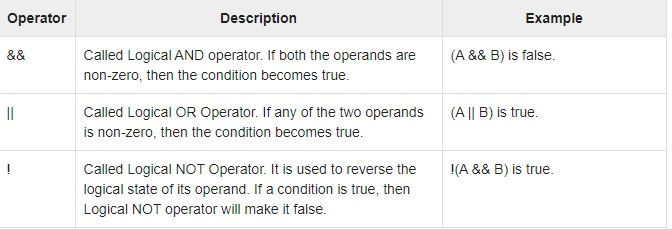
Bitwise Operators
Bitwise operator works on bits and performs bit-by-bit operation. The truth tables for &, |, and ^ is as follows −
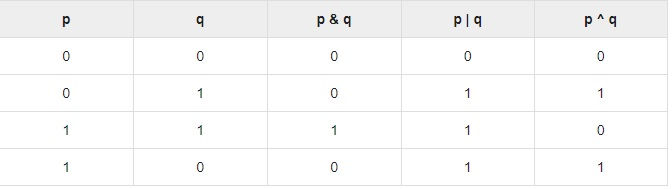
Assume A = 60 and B = 13 in binary format, they will be as follows −
A = 0011 1100
B = 0000 1101
-----------------
A&B = 0000 1100
A|B = 0011 1101
A^B = 0011 0001
~A = 1100 0011
The following table lists the bitwise operators supported by C. Assume variable 'A' holds 60 and variable 'B' holds 13, then −
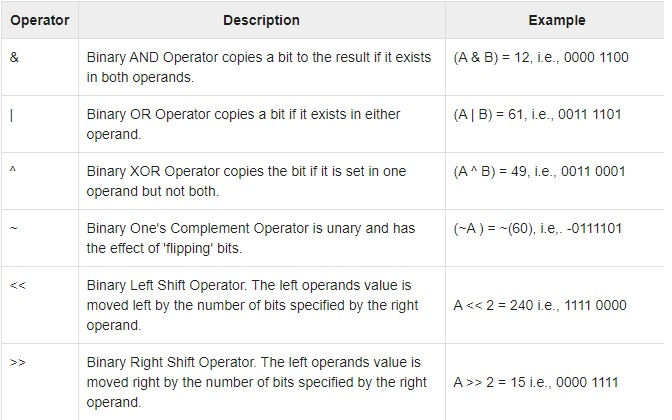
Assignment Operators
The following table lists the assignment operators supported by the C language −
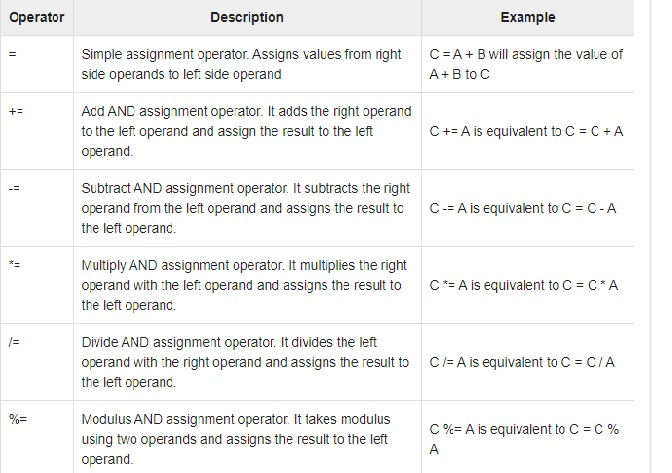

Misc Operators ↦ sizeof & ternary
Besides the operators discussed above, there are a few other important operators including sizeof and ? : supported by the C Language.
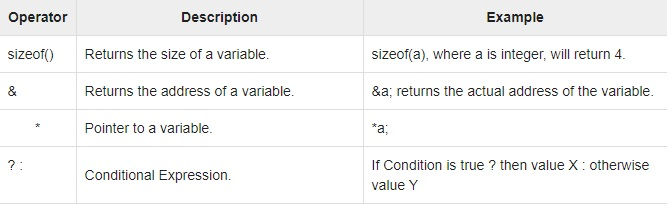
Operators Precedence in C
Operator precedence determines the grouping of terms in an expression and decides how an expression is evaluated. Certain operators have higher precedence than others; for example, the multiplication operator has a higher precedence than the addition operator.
For example, x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator * has a higher precedence than +, so it first gets multiplied with 3*2 and then adds into 7.
Here, operators with the highest precedence appear at the top of the table, those with the lowest appear at the bottom. Within an expression, higher precedence operators will be evaluated first.
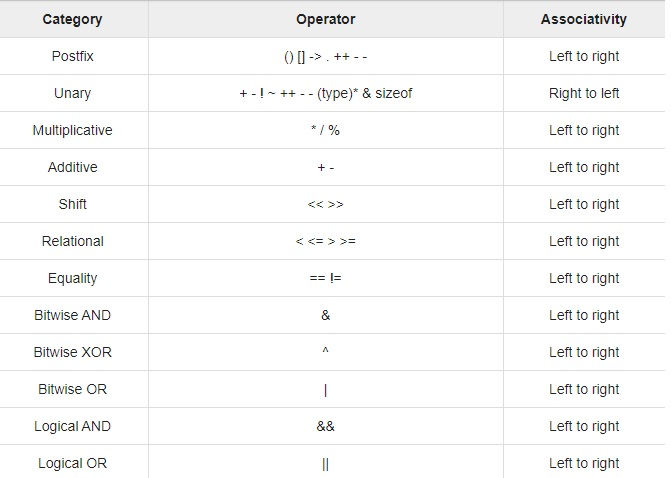
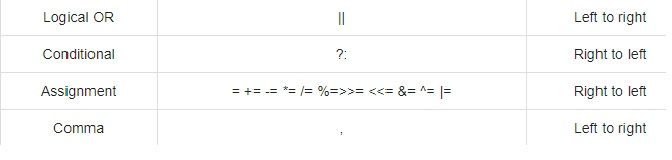
Increment and Decrement Operator in C
Increment Operators are used to increasing the value of the variable by one and Decrement Operators are used to decrease the value of the variable by one in C programs.
Both increment and decrement operator are used on a single operand or variable, so it is called as a unary operator. Unary operators are having a higher priority than the other operators it means unary operators are executed before other operators.
++ // increment operator
-- // decrement operator
Note: Increment and decrement operators are can not apply on constant.
x= 4++; // gives error, because 4 is constant
Type of Increment Operator
pre-incrementpost-increment
pre-increment (++ variable)
In pre-increment first increment the value of variable and then used inside the expression (initialize into another variable).
++ variable;
#include<stdio.h>
void main()
{
int x,i;
i=10;
x=++i;
printf("x: %d",x);
printf("i: %d",i);
getch();
}
x: 11
i: 11
In above program first increase the value of i and then used value of i into expression.
post-increment (variable ++)
In post-increment first value of variable is used in the expression (initialize into another variable) and then increment the value of variable.
variable ++;
#include<stdio.h>
void main()
{
int x,i;
i=10;
x=i++;
printf("x: %d",x);
printf("i: %d",i);
getch();
}
x: 10
i: 11
In above program first used the value of i into expression then increase value of i by 1.
Type of Decrement Operator
pre-decrementpost-decrement
Pre-decrement (-- variable)
In pre-decrement first decrement the value of variable and then used inside the expression (initialize into another variable).
-- variable;
#include<stdio.h>
void main()
{
int x,i;
i=10;
x=--i;
printf("x: %d",x);
printf("i: %d",i);
getch();
}
x: 9
i: 9
In above program first decrease the value of i and then value of i used in expression.
post-decrement (variable --)
In Post-decrement first value of variable is used in the expression (initialize into another variable) and then decrement the value of variable.
variable --;
#include<stdio.h>
void main()
{
int x,i;
i=10;
x=i--;
printf("x: %d",x);
printf("i: %d",i);
getch();
}
MCQ on Operators
1. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int i = -3;
int k = i % 2;
printf("%d\n", k);
}
a) Compile time error
b) -1
c) 1
d) Implementation defined
2. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int i = 3;
int l = i / -2;
int k = i % -2;
printf("%d %d\n", l, k);
return 0;
}
a) Compile time error
b) -1 1
c) 1 -1
d) Implementation defined
3. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int i = 5;
i = i / 3;
printf("%d\n", i);
return 0;
}
a) Implementation defined
b) 1
c) 3
d) Compile time error
4. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int i = -5;
i = i / 3;
printf("%d\n", i);
return 0;
}
a) Implementation defined
b) -1
c) -3
d) Compile time error
5. What will be the final value of x in the following C code?
#include <stdio.h>
void main()
{
int x = 5 * 9 / 3 + 9;
}
a) 3.75
b) Depends on compiler
c) 24
d) 3
6. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int x = 5.3 % 2;
printf("Value of x is %d", x);
}
a) Value of x is 2.3
b) Value of x is 1
c) Value of x is 0.3
d) Compile time error
7. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int y = 3;
int x = 5 % 2 * 3 / 2;
printf("Value of x is %d", x);
}
a) Value of x is 1 b) Value of x is 2 c) Value of x is 3 d) Compile time error
1. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int a = 3;
int b = ++a + a++ + --a;
printf("Value of b is %d", b);
}
a) Value of x is 12
b) Value of x is 13
c) Value of x is 10
d) Undefined behaviour
2. What is the precedence of arithmetic operators (from highest to lowest)?
a) %, *, /, +, –
b) %, +, /, *, –
c) +, -, %, *, /
d) %, +, -, *, /
3. Which of the following is not an arithmetic operation?
a) a * = 10;
b) a / = 10;
c) a ! = 10;
d) a % = 10;
4. Which of the following data type will throw an error on modulus operation(%)?
a) char
b) short
c) int
d) float
5. Which among the following are the fundamental arithmetic operators, i.e, performing the desired operation can be done using that operator only?
a) +, –
b) +, -, %
c) +, -, *, /
d) +, -, *, /, %
6. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int a = 10;
double b = 5.6;
int c;
c = a + b;
printf("%d", c);
}
a) 15
b) 16
c) 15.6
d) 10
7. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int a = 10, b = 5, c = 5;
int d;
d = a == (b + c);
printf("%d", d);
}
a) Syntax error b) 1 c) 10 d) 5
1. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int x = 1, y = 0, z = 5;
int a = x && y || z++;
printf("%d", z);
}
a) 6
b) 5
c) 0
d) Varies
2. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int x = 1, y = 0, z = 5;
int a = x && y && z++;
printf("%d", z);
}
a) 6
b) 5
c) 0
d) Varies
3. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 1, y = 0, z = 3;
x > y ? printf("%d", z) : return z;
}
a) 3
b) 1
c) Compile time error
d) Run time error
4. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int x = 1, z = 3;
int y = x << 3;
printf(" %d\n", y);
}
a) -2147483648
b) -1
c) Run time error
d) 8
5. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int x = 0, y = 2, z = 3;
int a = x & y | z;
printf("%d", a);
}
a) 3
b) 0
c) 2
d) Run time error
6. What will be the final value of j in the following C code?
#include <stdio.h>
int main()
{
int i = 0, j = 0;
if (i && (j = i + 10))
//do something
;
}
a) 0
b) 10
c) Depends on the compiler
d) Depends on language standard
7. What will be the final value of j in the following C code?
#include <stdio.h>
int main()
{
int i = 10, j = 0;
if (i || (j = i + 10))
//do something
;
}
a) 0
b) 20
c) Compile time error
d) Depends on language standard
8. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int i = 1;
if (i++ && (i == 1))
printf("Yes\n");
else
printf("No\n");
}
a) Yes b) No c) Depends on the compiler d) Depends on the standard
1. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int c = 2 ^ 3;
printf("%d\n", c);
}
a) 1
b) 8
c) 9
d) 0
2. What will be the output of the following C code?
#include <stdio.h>
int main()
{
unsigned int a = 10;
a = ~a;
printf("%d\n", a);
}
a) -9
b) -10
c) -11
d) 10
3. What will be the output of the following C code?
#include <stdio.h>
int main()
{
if (7 & 8)
printf("Honesty");
if ((~7 & 0x000f) == 8)
printf("is the best policy\n");
}
a) Honesty is the best policy
b) Honesty
c) is the best policy
d) No output
4. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int a = 2;
if (a >> 1)
printf("%d\n", a);
}
a) 0
b) 1
c) 2
d) No Output
5. Comment on the output of the following C code.
#include <stdio.h>
int main()
{
int i, n, a = 4;
scanf("%d", &n);
for (i = 0; i < n; i++)
a = a * 2;
}
a) Logical Shift left
b) No output
c) Arithmetic Shift right
d) Bitwise exclusive OR
6. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int x = 97;
int y = sizeof(x++);
printf("x is %d", x);
}
a) x is 97
b) x is 98
c) x is 99
d) Run time error
7. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int x = 4, y, z;
y = --x;
z = x--;
printf("%d%d%d", x, y, z);
}
a) 3 2 3
b) 2 2 3
c) 3 2 2
d) 2 3 3
8. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int x = 4;
int *p = &x;
int *k = p++;
int r = p - k;
printf("%d", r);
}
a) 4 b) 8 c) 1 d) Run time error
1. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int x = 0;
if (x = 0)
printf("Its zero\n");
else
printf("Its not zero\n");
}
a) Its not zero
b) Its zero
c) Run time error
d) None
2. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int k = 8;
int x = 0 == 1 && k++;
printf("%d%d\n", x, k);
}
a) 0 9
b) 0 8
c) 1 8
d) 1 9
3. What will be the output of the following C code?
#include <stdio.h>
void main()
{
char a = 'a';
int x = (a % 10)++;
printf("%d\n", x);
}
a) 6
b) Junk value
c) Compile time error
d) 7
4. What will be the output of the following C code snippet?
#include <stdio.h>
void main()
{
1 < 2 ? return 1: return 2;
}
a) returns 1
b) returns 2
c) Varies
d) Compile time error
5. What will be the output of the following C code snippet?
#include <stdio.h>
void main()
{
unsigned int x = -5;
printf("%d", x);
}
a) Run time error
b) Aries
c) -5
d) 5
6. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 1;
x *= x + y;
printf("%d\n", x);
return 0;
}
a) 5
b) 6
c) Undefined behaviour
d) Compile time error
7. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 2;
x /= x / y;
printf("%d\n", x);
return 0;
}
a) 2
b) 1
c) 0.5
d) Undefined behaviour
8. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 1, y = 0;
x &&= y;
printf("%d\n", x);
}
a) Compile time error b) 1 c) 0 d) Undefined behaviour
1. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int x = 0;
if (x = 0)
printf("Its zero\n");
else
printf("Its not zero\n");
}
a) Its not zero
b) Its zero
c) Run time error
d) None
2. What will be the output of the following C code?
#include <stdio.h>
void main()
{
int k = 8;
int x = 0 == 1 && k++;
printf("%d%d\n", x, k);
}
a) 0 9
b) 0 8
c) 1 8
d) 1 9
3. What will be the output of the following C code?
#include <stdio.h>
void main()
{
char a = 'a';
int x = (a % 10)++;
printf("%d\n", x);
}
a) 6
b) Junk value
c) Compile time error
d) 7
4. What will be the output of the following C code snippet?
#include <stdio.h>
void main()
{
1 < 2 ? return 1: return 2;
}
a) returns 1
b) returns 2
c) Varies
d) Compile time error
5. What will be the output of the following C code snippet?
#include <stdio.h>
void main()
{
unsigned int x = -5;
printf("%d", x);
}
a) Run time error
b) Aries
c) -5
d) 5
6. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 1;
x *= x + y;
printf("%d\n", x);
return 0;
}
a) 5
b) 6
c) Undefined behaviour
d) Compile time error
7. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 2;
x /= x / y;
printf("%d\n", x);
return 0;
}
a) 2
b) 1
c) 0.5
d) Undefined behaviour
8. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 1, y = 0;
x &&= y;
printf("%d\n", x);
}
a) Compile time error b) 1 c) 0 d) Undefined behaviour
1. What will be the output of the following C function?
#include <stdio.h>
int main()
{
reverse(1);
}
void reverse(int i)
{
if (i > 5)
exit(0);
printf("%d\n", i);
return reverse(i++);
}
a) 1 2 3 4 5
b) 1 2 3 4
c) Compile time error
d) Stack overflow
2. What will be the output of the following C function?
#include <stdio.h>
void reverse(int i);
int main()
{
reverse(1);
}
void reverse(int i)
{
if (i > 5)
return ;
printf("%d ", i);
return reverse((i++, i));
}
a) 1 2 3 4 5
b) Segmentation fault
c) Compilation error
d) Undefined behaviour
3. In expression i = g() + f(), first function called depends on __________
a) Compiler
b) Associativiy of () operator
c) Precedence of () and + operator
d) Left to write of the expression
4. What will be the final values of i and j in the following C code?
#include <stdio.h>
int x = 0;
int main()
{
int i = (f() + g()) || g();
int j = g() || (f() + g());
}
int f()
{
if (x == 0)
return x + 1;
else
return x - 1;
}
int g()
{
return x++;
}
a) i value is 1 and j value is 1
b) i value is 0 and j value is 0
c) i value is 1 and j value is undefined
d) i and j value are undefined
5. What will be the final values of i and j in the following C code?
#include <stdio.h>
int x = 0;
int main()
{
int i = (f() + g()) | g(); //bitwise or
int j = g() | (f() + g()); //bitwise or
}
int f()
{
if (x == 0)
return x + 1;
else
return x - 1;
}
int g()
{
return x++;
}
a) i value is 1 and j value is 1
b) i value is 0 and j value is 0
c) i value is 1 and j value is undefined
d) i and j value are undefined
6. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 0;
int z = y && (y |= 10);
printf("%d\n", z);
return 0;
}
a) 1
b) 0
c) Undefined behaviour due to order of evaluation
d) 2
7. What will be the output of the following C code?
advertisement
#include <stdio.h>
int main()
{
int x = 2, y = 0;
int z = (y++) ? 2 : y == 1 && x;
printf("%d\n", z);
return 0;
}
a) 0
b) 1
c) 2
d) Undefined behaviour
8. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 0;
int z;
z = (y++, y);
printf("%d\n", z);
return 0;
}
a) 0
b) 1
c) Undefined behaviour
d) Compilation error
9. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int x = 2, y = 0, l;
int z;
z = y = 1, l = x && y;
printf("%d\n", l);
return 0;
}
a) 0
b) 1
c) Undefined behaviour due to order of evaluation can be different
d) Compilation error
10. What will be the output of the following C code?
#include <stdio.h>
int main()
{
int y = 2;
int z = y +(y = 10);
printf("%d\n", z);
}
a) 12 b) 20 c) 4 d) Either 12 or 20
Comments